以前、こちらの記事で記載しました、VisualStudioがなくてもC#をコンパイルする方法にて
僕が自分で作成した簡易ファイルランチャーのコード載せますよーという
お話をしておりました。
このコードはどちらかというと、自分のためというか・・・。
現場で仕事しているとセキュリティの都合上、
webサービスにログイン自体ができなかったりして
ソースコード達をいくらオンラインストレージなどに固めていても
ダウンロード出来ければ意味がありません。
ブログで調べているという体なら、
自分のコードたちをコピーすればいいわけですから
やりやすいということになると考えたんです。
と、いうことで↓にファイル単位に説明は軽く載せていきますー。
MyLauncher.cs
これはメインメニュー部にあたります。
using System; using System.Collections.Generic; using System.CodeDom; using System.CodeDom.Compiler; using System.Reflection; using System.Windows.Forms; using System.Drawing; using System.ComponentModel; using System.Runtime.InteropServices; public class MyLauncher : BaseForm { [STAThread] public static void Main() { GeneratedCodeAttribute generatedCodeAttribute = new GeneratedCodeAttribute( "Microsoft.VisualStudio.Editors.SettindsDesigner.SettingsSingleFileGenerator", "10.0.0.0" ); Application.EnableVisualStyles(); Application.SetCompatibleTextRenderingDefault(false); Application.Run( new MyLauncher() ); } public MyLauncher() { // 初期画面なのでトップに戻るボタンを削除 removeGoTop(); // Dropbox Controls.Add(new MyButton(1, 1, "Dropbox", delegate(object sender, System.EventArgs e) { System.Diagnostics.Process.Start(@"Dropboxフォルダ"); })); // アプリケーション Controls.Add(new MyButton(1, 2, "アプリケーション", delegate(object sender, System.EventArgs e) { new MyLauncherApplications().Show(this); }, false)); } } public class MyLauncherApplications : BaseForm { public MyLauncherApplications() { // foobar2000 Controls.Add(new MyButton(1, 1, "", delegate(object sender, System.EventArgs e) { System.Diagnostics.Process.Start(@"C:\test\foobar2000.exe"); }, @"C:\test\foobar2000.exe")); // Excel資料 Controls.Add(new MyButton(2, 1, "管理ファイル", delegate(object sender, System.EventArgs e) { Utils.startWithWaiting(@"C:\Test]\管理.xlsx", "Microsoft Excel - 管理.xlsx"); })); } }
MyLauncherParts.cs
これは自前で拡張した部品類にあたります。
using System; using System.Collections.Generic; using System.CodeDom; using System.CodeDom.Compiler; using System.Reflection; using System.Windows.Forms; using System.Drawing; using System.ComponentModel; using System.Runtime.InteropServices; public class MyButton : Button { public MyButton(Int32 x, Int32 y, String caption, EventHandler eventMethod) { setConfig(x, y, caption, eventMethod, null, true); } public MyButton(Int32 x, Int32 y, String caption, EventHandler eventMethod, bool isMinimized) { setConfig(x, y, caption, eventMethod, null, isMinimized); } public MyButton(Int32 x, Int32 y, String caption, EventHandler eventMethod, String iconPath) { setConfig(x, y, caption, eventMethod, iconPath, true); } public MyButton(Int32 x, Int32 y, String caption, EventHandler eventMethod, String iconPath, bool isMinimized) { setConfig(x, y, caption, eventMethod, iconPath, isMinimized); } private void setConfig(Int32 x, Int32 y, String caption, EventHandler eventMethod, String iconPath, bool isMinimized) { // x:画面左から何列目のボタンか // y:画面上から何行目のボタンか Int32 xPoint = ((x - 1) * (Utils.buttonWidth - 1)) - (x - 1); Int32 yPoint = ((y - 1) * (Utils.buttonHeight - 1)) - (y - 1); this.Location = new Point(xPoint, yPoint); this.Size = Utils.getButtonSize(); this.Click += eventMethod; if (isMinimized) { this.Click += delegate(object sender, EventArgs e) { new MyLauncher().Show(Utils.currentForm); Utils.currentForm.WindowState = FormWindowState.Minimized; }; } if (caption != null) { this.Text = caption; } if (iconPath != null) { this.Image = System.Drawing.Icon.ExtractAssociatedIcon(iconPath).ToBitmap(); } } } public class BaseForm : Form { public Button goTopButton; public BaseForm() { Text = "My Launcher"; StartPosition = FormStartPosition.Manual; Location = Utils.getStartPosition(); ClientSize = Utils.getFormSize(); this.TopMost = true; this.Load += delegate(object sender, System.EventArgs e) { Utils.currentForm = this; Utils.unRegistHotKey(); Utils.handle = this.Handle; Utils.registHotKey(); }; this.Closed += delegate(object sender2, System.EventArgs e2) { Utils.unRegistHotKey(); Application.Exit(); }; Controls.Add(Utils.getAppearDesktopFolderButton()); Controls.Add(Utils.getAppearDesktopButton()); goTopButton = Utils.getGoTopButton(this); Controls.Add(goTopButton); Utils.setIcon(this); } public void Show(BaseForm f) { this.Show(); f.Hide(); } public void removeGoTop() { Controls.Remove(goTopButton); } protected override void WndProc(ref Message m) { base.WndProc(ref m); if (m.Msg == Utils.WM_HOTKEY) { if ((int)m.WParam == Utils.HOTKEY_ID) { if (this.WindowState == FormWindowState.Minimized) { this.WindowState = FormWindowState.Normal; } else { this.WindowState = FormWindowState.Minimized; } } } } } public class WaitingForm : Form { public WaitingForm() { Text = "起動中・・・・・"; StartPosition = FormStartPosition.CenterScreen; ClientSize = new Size(200, 100); Label label = new Label(); label.Text = "起動中・・・"; label.AutoSize = true; label.Location = new Point(0, 40); Controls.Add(label); } }
MyLauncherUtils.cs
これはユーティリティー(小部品群)にあたります。
using System; using System.Collections.Generic; using System.CodeDom; using System.CodeDom.Compiler; using System.Reflection; using System.Windows.Forms; using System.Drawing; using System.ComponentModel; using System.Runtime.InteropServices; class Utils { public static IntPtr handle; public static BaseForm currentForm; public const int MOD_ALT = 0x0001; public const int MOD_CONTROL = 0x0002; public const int MOD_SHIFT = 0x0004; public const int WM_HOTKEY = 0x0312; public const int HOTKEY_ID = 0x0001; public const int HOTKEY2_ID = 0x0002; [DllImport("user32.dll")] static extern int RegisterHotKey(IntPtr HWnd, int ID, int MOD_KEY, int KEY); [DllImport("user32.dll")] static extern int UnregisterHotKey(IntPtr HWnd, int ID); [DllImport("shell32.dll", CharSet = CharSet.Unicode)] static extern void SHGetStockIconInfo(UInt32 siid, UInt32 uFlags, ref SHSTOCKICONINFO sii); // 接続切断するWin32 APIを宣言 [DllImport("mpr.dll", EntryPoint = "WNetCancelConnection2", CharSet = CharSet.Unicode)] static extern int WNetCancelConnection2(string lpName, Int32 dwFlags, bool fForce); // 認証情報を使用して接続するWin32 APIを宣言 [DllImport("mpr.dll", EntryPoint = "WNetAddConnection2", CharSet = CharSet.Unicode)] static extern int WNetAddConnection2(ref NETRESOURCE lpNetResource, string lpPassword, string lpUsername, Int32 dwFlags); [StructLayoutAttribute(LayoutKind.Sequential, CharSet = CharSet.Unicode)] struct SHSTOCKICONINFO { public Int32 cbSize; public IntPtr hIcon; public Int32 iSysImageIndex; public Int32 iIcon; [MarshalAs(UnmanagedType.ByValTStr, SizeConst = 260)] public string szPath; } // WNetAddConnection2に渡す接続の認証情報の構造体 [StructLayout(LayoutKind.Sequential)] internal struct NETRESOURCE { public int dwScope; public int dwType; public int dwDisplayType; public int dwUsage; [MarshalAs(UnmanagedType.LPWStr)] public string lpLocalName; [MarshalAs(UnmanagedType.LPWStr)] public string lpRemoteName; [MarshalAs(UnmanagedType.LPWStr)] public string lpComment; [MarshalAs(UnmanagedType.LPWStr)] public string lpProvider; } public const UInt32 SHGSI_ICON = 0x000000100; public const UInt32 SHGSI_SMALLICON = 0x000000001; public const UInt32 SIID_SHIELD = 0x00000004D; public const Int32 buttonWidth = 100; public const Int32 buttonHeight = 50; public const Int32 appearDeskTopButtonPosX = 5; public const Int32 appearDeskTopButtonPosY = 3; public const Int32 appearDeskTopButtonFolderPosX = 4; public const Int32 appearDeskTopButtonFolderPosY = 3; public const Int32 goTopPosX = 3; public const Int32 goTopPosY = 3; public static Size getButtonSize() { return new Size(buttonWidth, buttonHeight); } public static Size getFormSize() { return new Size(493, 146); } public static Point getStartPosition() { return new Point(77, 0); } public static Button getAppearDesktopButton() { return getAppearDesktopButton(appearDeskTopButtonPosX, appearDeskTopButtonPosY); } public static Button getAppearDesktopButton(Int32 x, Int32 y) { // デスクトップを表示 return new MyButton(x, y, "デスクトップの表示", delegate(object sender, System.EventArgs e) { System.Diagnostics.Process.Start("exploer.exe", "shell:::{3080F90D-D7AD-11D9-BD98-0000947B0257}"); }); } public static Button getAppearDesktopFolderButton() { return getAppearDesktopFolderButton(appearDeskTopButtonFolderPosX, appearDeskTopButtonFolderPosY); } public static Button getAppearDesktopFolderButton(Int32 x, Int32 y) { // デスクトップを表示 return new MyButton(x, y, "デスクトップ\nフォルダ", delegate(object sender, System.EventArgs e) { System.Diagnostics.Process.Start("exploer.exe", @"C:\Users\User\Desktop"); }); } public static Button getGoTopButton(BaseForm form) { return getGoTopButton(goTopPosX, goTopPosY, form); } public static Button getGoTopButton(Int32 x, Int32 y, BaseForm form) { return new MyButton(x, y, "トップへ戻る", delegate(object sender, System.EventArgs e) { new MyLauncher().Show(form); }, false); } public static void connectNwDir(String dir) { try { System.Diagnostics.Process.Start(dir); } catch (Exception) { // 接続情報を設定 NETRESOURCE netResource = new NETRESOURCE(); netResource.dwScope = 0; netResource.dwType = 1; netResource.dwDisplayType = 0; netResource.dwUsage = 0; netResource.lpLocalName = ""; // ネットワークドライブの場合は、ドライブレター netResource.lpRemoteName = dir; netResource.lpProvider = ""; string password = "pass"; string userId = "id"; try { // 一旦切断 WNetCancelConnection2(dir, 0 , true); // 接続 WNetAddConnection2(ref netResource, password, userId, 0); System.Diagnostics.Process.Start(dir); } catch (Exception e1) { throw e1; } } } public static void startWithWaiting(String exePath, String searchTask) { WaitingForm f = new WaitingForm(); f.Show(); System.Diagnostics.Process.Start(exePath); DateTime start = DateTime.Now; DateTime end = start.AddMinutes(1); bool flg = true; while(flg) { foreach(System.Diagnostics.Process p in System.Diagnostics.Process.GetProcesses()) { if (DateTime.Now > end) { MessageBox.Show("起動に時間がかかっています"); f.Hide(); return; } if (p.MainWindowHandle != IntPtr.Zero) { if (searchTask.Equals(p.MainWindowTitle)) { flg = false; } } } } f.Hide(); } public static void setIcon(BaseForm form) { SHSTOCKICONINFO sii = new SHSTOCKICONINFO(); sii.cbSize = Marshal.SizeOf(sii); SHGetStockIconInfo(SIID_SHIELD, SHGSI_ICON | SHGSI_SMALLICON, ref sii); if (sii.hIcon != IntPtr.Zero) { form.Icon = Icon.FromHandle(sii.hIcon); } } public static void registHotKey() { RegisterHotKey(handle, HOTKEY_ID, 0, (int)Keys.F1); } public static void unRegistHotKey() { UnregisterHotKey(handle, HOTKEY_ID); } }
最後に
これらをこちらで記載したやり方でコンパイルすると
こんな感じのランチャーが出来上がりです。
コードを見ていただくと色々なことを実はやっていたりしますが、
そのそれぞれの内容については希望があれば解説したいと思います。
それでは!
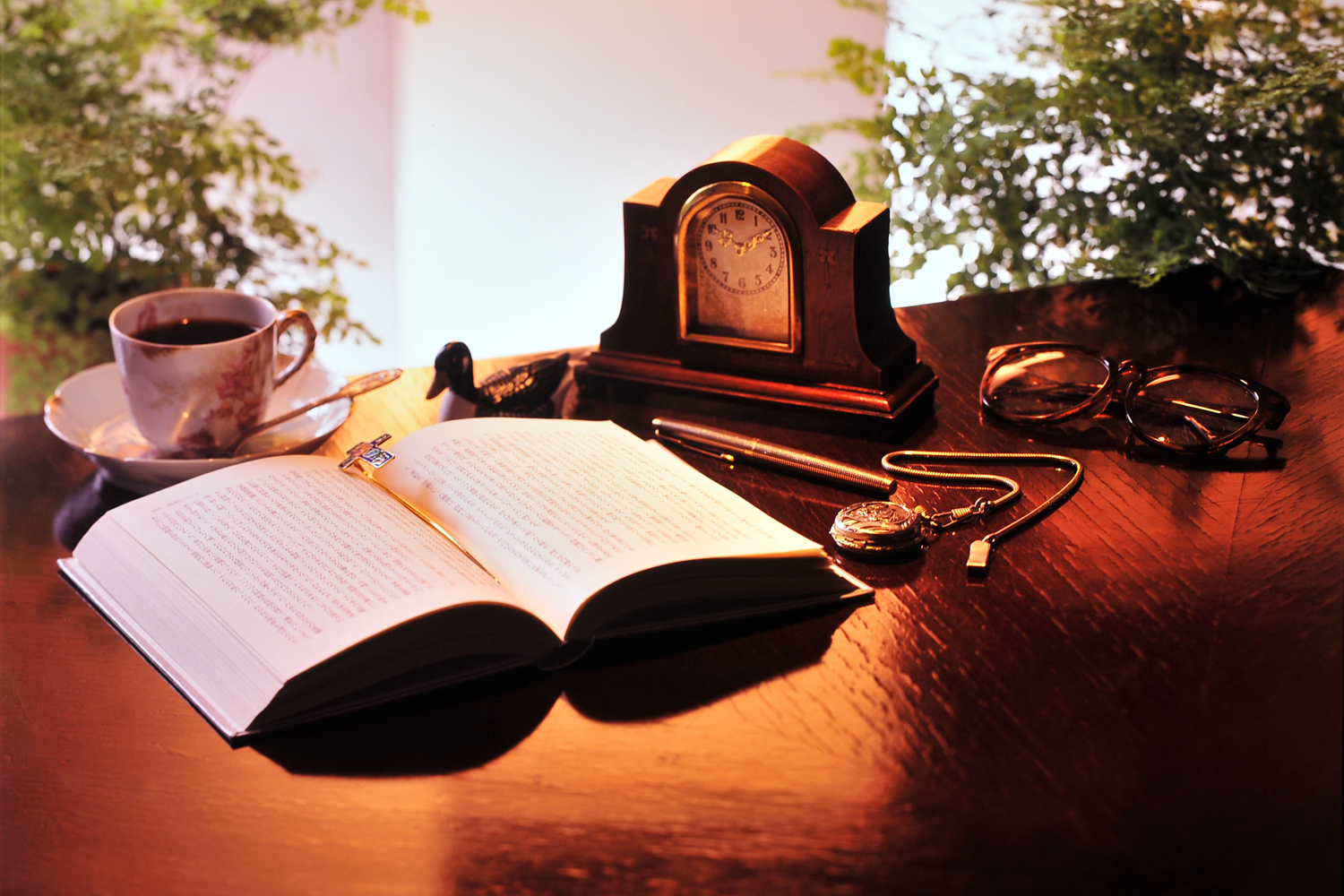
20代前半までは東京で音楽をやりながら両手の指以上の業種でアルバイト生活をしていましたが、某大手プロバイダのテレアポのバイトでPCの知識の無さに愕然とし、コンピュータをもっと知りたい!と思ったことをきっかけに25歳の時にITの世界に未経験で飛び込みました。
紆余曲折を経て、現在は個人事業主としてお仕事させていただいており、10年ほどになります。
web制作から企業システム構築、ツール開発など、フロントエンドもバックエンドもサーバーもDBAも依頼があれば何でもやってきた雑食系エンジニアです。
今風にいうとフルスタックエンジニアということになるのでしょうか??
→ 詳細プロフィールというか、生い立ちはこちら
→スキルシートをご覧になる場合はこちら
→お仕事のご依頼やお見積りなどお問い合わせはこちらから!
コメント